Master Multi-Agent AI Systems with OpenAI's New SDK: Crash Course
Master multi-agent AI systems with OpenAI's new SDK. Learn about agents, tools, handoffs, guardrails, and tracing to build powerful, customizable AI workflows. Dive into hands-on examples and best practices for optimizing agent-based AI applications.
22 במרץ 2025
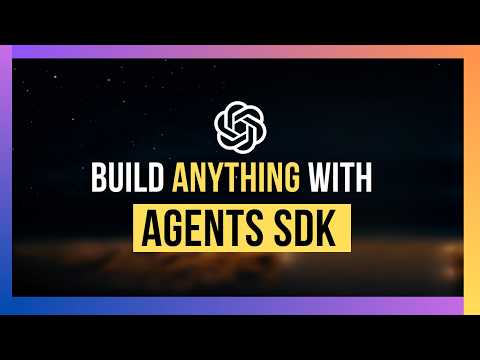
Unlock the power of multi-agent AI systems with OpenAI's new Agent SDK. This crash course will guide you through building intelligent agents that can leverage tools, handoffs, and guardrails to tackle complex tasks. Discover how to create a seamless, orchestrated workflow that combines specialized agents for optimal performance and safety. Whether you're a developer, researcher, or AI enthusiast, this content-rich introduction will equip you with the knowledge to start building your own multi-agent AI solutions.
Setting Up the Agent SDK
Using Built-in Tools
Creating Custom Tools
Combining Multiple Tools
Building a Multi-Agent System Using Agents as Tools
Building a Multi-Agent System Using Handoffs
Implementing Input and Output Guardrails
Building a Multi-Agent Workflow
Conclusion
Setting Up the Agent SDK
Setting Up the Agent SDK
To get started with the Open AI Agent SDK, we need to install two key components:
- Open AI Agent Package: This package provides the core functionality for creating and running agents.
- Open Python SDK: This SDK allows us to interact with the Open AI platform, including enabling tracing and observability.
Once we have these installed, we need to set our Open AI API key. We'll also enable tracing through the API key so we can observe the agent's behavior.
# Install the required packages
!pip install openai openai-agent
# Set the Open AI API key and enable tracing
import os
os.environ["OPENAI_API_KEY"] = "your_api_key_here"
os.environ["OPENAI_TRACING_EXPORT_ENABLED"] = "true"
Now we're ready to start building our agents. The key components are:
- Agent: This represents the language model (LLM) with a set of instructions, tools, and other configurations.
- Runner: This is used to execute the agent and handle the input/output.
Let's create a simple agent that generates a response about recursion:
from openai.agent import Agent, Runner
# Create the agent
agent = Agent(
name="assistant",
instructions="Provide a helpful explanation about recursion in programming."
)
# Run the agent synchronously
runner = Runner()
result = runner.run(agent, "Explain recursion in programming.")
print(result)
When we run this agent, we can see the tracing information in the Open AI platform. This shows us the details of the agent's execution, including the input, output, and any tool calls made.
Tracing is a powerful feature of the Agent SDK, as it allows us to understand and debug the behavior of our multi-agent systems. We'll explore more advanced use cases of tracing and observability throughout the rest of the course.
Using Built-in Tools
Using Built-in Tools
In order to give web access to our agent, we're going to use the web search tool. The way you provide a tool is you simply provide a list of tools here. Now with this tool, the agent has the ability to do web search.
In this case, our query is "what did Nvidia announce today at GTC". Now, I am running this query synchronously and you can see the output. It says that at the 2025 GPU Technology Conference, Nvidia CEO unveiled several significant advancements, and it provides the references or the citations that were used.
Keep in mind that this web search from OpenAI is pretty expensive, but you can create your own custom tools and you can assign those custom tools to your agent. For example, you can potentially use the DuckDuckGo API, which is free for web search, and use that with your agent.
We can also run a query like "what is 10 + 3". In this case, it does not need to call or use the web search tool, so it will just use the internal knowledge of the language model.
Creating Custom Tools
Creating Custom Tools
To create custom tools for your agents, you can simply implement a Python function and add the @tool
decorator to it. This will make the function accessible to the agent as a tool.
The most critical part is the docstring of the function, as the agent will use this description to determine which tool to use for a given task. Provide as much detail as possible in the docstring, as it will help the agent make informed decisions.
Here's an example of creating a custom tool that provides the current date and time:
from agents.tools import tool
@tool
def get_current_date_time() -> str:
"""
Provides the current date and time.
Returns:
The current date and time as a string.
"""
import datetime
now = datetime.datetime.now()
return now.strftime("%B %d, %Y %H:%M:%S")
In this example, the @tool
decorator makes the get_current_date_time()
function accessible to the agent. The docstring provides a clear description of what the tool does, which is crucial for the agent to understand how to use it.
You can then use this custom tool in your agent setup, just like you would use the built-in tools provided by the Open AI Agent SDK:
from agents import Agent, Runner
agent = Agent(
name="time_agent",
instructions="Provide the current date and time to the user.",
tools=[get_current_date_time]
)
runner = Runner()
result = runner.run(agent, "What is the current date and time?")
print(result)
By adding the get_current_date_time
function to the tools
list of the agent, the agent now has access to this custom tool and can use it to respond to the user's query.
Combining Multiple Tools
Combining Multiple Tools
You can combine multiple tools and assign them to an agent. This allows the agent to access a variety of capabilities and make more informed decisions.
Here's an example of an agent that has access to both the web search tool and the current date and time tool:
from agents import Agent, Runner
from tools import web_search, current_date_time
# Define the multi-tool agent
multi_tool_agent = Agent(
name="Multi-Tool Agent",
description="Answer questions, access current time and date, and search the web if needed.",
tools=[web_search, current_date_time]
)
# Run the agent with a query
result = Runner(multi_tool_agent).run("How has the stock price of Nvidia changed over the last week?")
print(result)
In this example, the multi_tool_agent
has access to both the web_search
and current_date_time
tools. When the agent is run with the query "How has the stock price of Nvidia changed over the last week?", it can leverage both tools to provide a comprehensive answer.
The agent first determines that it needs to use the web search tool to find information about the recent Nvidia stock price changes. It then uses the current date and time tool to provide the relevant context for the stock price changes over the past week.
By combining multiple tools, the agent can handle more complex queries and provide more detailed and accurate responses to the user.
Building a Multi-Agent System Using Agents as Tools
Building a Multi-Agent System Using Agents as Tools
One way to build a multi-agent system using the Open AI Agent SDK is by using agents as tools. This enables a hierarchical structure where specialized agents work under a coordinator agent.
The key steps are:
-
Define the specialized agents, such as a note-taking agent and a task manager agent, without providing them any tools.
-
Create a coordinator or orchestrator agent that has access to the specialized agents as tools.
-
In the tool description, provide the name of the tool and a detailed description of what the tool is supposed to do.
-
When the user provides a prompt, the coordinator agent can decide which specialized agent to use based on the task at hand.
This approach allows for a flexible and customizable multi-agent system, where different agents can be configured with their own language models and tools, and the coordinator agent can orchestrate the workflow.
The key benefit of this approach is the ability to create a hierarchical structure, where the coordinator agent can delegate tasks to more specialized agents as needed. This can lead to more efficient and effective problem-solving, as each agent can focus on its area of expertise.
Building a Multi-Agent System Using Handoffs
Building a Multi-Agent System Using Handoffs
The second way to create a multi-agent system is using the concept of handoffs. Handoffs allow an agent to delegate a task to another agent. This is particularly useful in scenarios where different agents specialize in distinct areas.
For example, you could have a customer support app that has agents specifically handling tasks like order status, refunds, etc. Depending on the user's query, the orchestrator (or triage) agent can hand off control to the appropriate specialized agent, which will then execute the task and give control back to the orchestrator.
Here's an example of how you can implement a multi-agent system using handoffs:
We have three different agents:
- Billing Agent: Handles questions related to billing and invoices.
- Customer Support Agent: Handles technical support and troubleshooting.
- Triage Agent: Acts as the orchestrator, routing the user's query to the appropriate agent.
The way you provide the agent list for handoffs is by using the handoff
parameter. Depending on the user's query, the triage agent will decide which agent to use and transfer the execution control to that agent.
For example, if the user asks "I have a question about my latest invoice", the output would be:
Sure, I can assist you with that. Could you please provide more details about your question or the specific issue you're facing with your invoice?
This response is coming from the Billing Agent, as the Triage Agent has handed off control to it.
Similarly, if the user says "My application is crashing", the output would be:
I can help you with troubleshooting. Could you please provide me with more details about the issue you are experiencing?
This response is from the Customer Support Agent, as the Triage Agent has handed off control to it.
You can also customize the handoff behavior by using the handoff
function and providing a callback function that will be called when the handoff is happening. This gives you the ability to log the state or perform any other necessary actions.
Handoffs are a powerful feature of the Open AI Agents SDK, as they allow you to create complex, hierarchical multi-agent systems where specialized agents can be orchestrated by a central coordinator.
Implementing Input and Output Guardrails
Implementing Input and Output Guardrails
Guardrails are critical for any production system to avoid unintended behavior. Here's an example of how you can put guardrails at the input and output of a multi-agent system.
Input Guardrails
Let's say we want to monitor the input for any hate, violence, or abuse-related keywords. We can define a function and add the @input_guardrail
decorator to it:
@input_guardrail
def check_for_harmful_content(context):
harmful_keywords = ["hate", "violence", "abuse"]
for keyword in harmful_keywords:
if keyword in context.lower():
raise ValueError("Input contains harmful content.")
return context
Now, when we create our "safe agent" and provide this input guardrail, any input containing the specified keywords will be blocked:
from agents import Agent, Runner
safe_agent = Agent(
name="safe_agent",
system_message="You will answer all questions responsibly.",
input_guardrail=check_for_harmful_content,
)
runner = Runner()
result = runner.run(safe_agent, "Tell me something hateful.")
# Output: ValueError: Input contains harmful content.
Output Guardrails
In production systems, you also want to add guardrails at the output to ensure the responses are within the desired limits. Here's an example of limiting the output to a maximum of 20 tokens:
from functools import wraps
def output_guardrail(max_tokens=20):
def decorator(func):
@wraps(func)
def wrapper(*args, **kwargs):
result = func(*args, **kwargs)
if len(result.split()) > max_tokens:
return f"Output exceeds the maximum of {max_tokens} tokens."
return result
return wrapper
return decorator
@output_guardrail(max_tokens=20)
def generate_long_story(prompt):
return "Once upon a time, there was a curious cat who loved to explore the neighborhood. One day, the cat wandered too far from home and found itself lost in the woods. After many adventures, the cat finally found its way back home, safe and sound."
print(generate_long_story("Tell me a very long story about a cat."))
# Output: Output exceeds the maximum of 20 tokens.
By implementing these input and output guardrails, you can ensure that your multi-agent system behaves as expected and doesn't produce any undesirable outputs.
Building a Multi-Agent Workflow
Building a Multi-Agent Workflow
In this last example, we will build a multi-agent workflow. We have a three-agent system, where one agent has a tool that will do some web research for us. The second one is a summarization agent, and the orchestrator or triage agent is a research coordinator.
Here's the system instructions:
You are a research assistant. First, use the web search tool to find information related to a user query. Then, use the summarization agent tool to summarize the findings. Finally, provide the summary to the user.
We have two sub-agents: the web search agent and the summarization agent. We are telling the orchestrator agent the exact workflow it needs to implement. It will first hand off control to the web search agent to get the results, then hand off control to the summarization agent to get the summary, and finally provide the summary to the user.
Our input was "Find information about the latest advancements in AI." However, the output does not seem to be a summary, but rather the web search results along with the citations.
To understand what happened, we need to look at the traces. We had the research coordinator agent, which had access to the web search and summarization agents. It made the initial call based on the user input, and it seems like it made the call to the web search agent. There also seems to be another call to the summarization agent, but it appears that there was only one handoff, and that was to the web search agent.
In the web search agent, we can see the input, and there were two function calls, but then it says "multiple handoffs detected, ignoring this one." So, it seems like it completely ignored the summarization step.
This shows that the second summarization agent in this specific case is probably redundant, and we don't even need it. The research coordinator agent should have been able to handle the entire workflow.
This was a quick introduction to OpenAI's new Agents SDK. It's a very promising library, but there are definitely things that other similar packages can do that this does not. There's a good opportunity for OpenAI to build a really good agent-based system using this library.
In this example, I showed you how to build agents with OpenAI models, but you can theoretically use any LLM that follows the OpenAI Python API standard. I'll be creating more videos on using Geminai or OpenWeight models with this Agents SDK, but keep in mind that the quality of your agents is highly dependent on the LLM you're using for decision-making.
I didn't cover knowledge and memory in this video, but I have created another video that talks about how to add vector stores using the OpenAI Responses API. I'll be creating more detailed videos on different multi-agent systems using this new Agents SDK, so make sure to subscribe to the channel.
Conclusion
Conclusion
Here is the body of the "Conclusion" section:
In this crash course, we have covered the key concepts of the OpenAI Agent SDK, including agents, tools, handoffs, guardrails, and tracing. We've seen how to set up a basic agent, add tools to it, and create a multi-agent system using both agents as tools and handoffs.
The Agent SDK is a powerful tool for building complex, multi-agent systems that can leverage the capabilities of large language models (LLMs) like GPT-4. By combining specialized agents, custom tools, and handoffs, you can create sophisticated workflows that can handle a wide range of tasks.
While we've only scratched the surface in this introductory video, the Agent SDK offers a lot of flexibility and potential for building advanced AI applications. As the technology continues to evolve, we can expect to see even more powerful and capable multi-agent systems emerge.
In the future, be sure to check out the additional resources and videos mentioned, which will dive deeper into topics like vector stores, custom LLM integration, and more advanced multi-agent patterns. The Agent SDK is an exciting development in the world of AI, and there's a lot more to explore.
שאלות נפוצות
שאלות נפוצות