Mastering MCP Server Setup and Tool Integration for Agents
Mastering MCP Server Setup and Tool Integration for Agents: Learn how to set up an MCP server, create a custom tool, and integrate it seamlessly into the Claude desktop assistant. Optimize for efficient AI assistant integration.
June 14, 2025
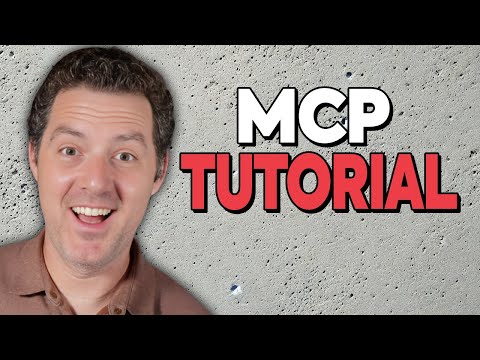
Discover how to effortlessly set up an MCP server and leverage powerful tools within your AI assistant or agent. This blog post provides a step-by-step guide, enabling you to seamlessly integrate custom tools and expand the capabilities of your AI-powered applications.
What is an MCP Server?
Setting up the MCP Server Environment
Creating the MCP Server
Configuring the MCP Server in Claude Desktop
Using the MCP Server Tool in Claude Desktop
Conclusion
What is an MCP Server?
What is an MCP Server?
An MCP (Micro-Controller Protocol) server is a tool that allows you to expose a set of tools or functionalities to an AI assistant or agent, such as Claude. It provides a way to integrate custom tools and services into the AI's capabilities, enabling you to extend its functionality beyond the default set of features.
The key aspects of an MCP server are:
-
Tool Exposure: The MCP server allows you to define and expose specific tools or functionalities that can be accessed by the AI assistant. These tools can be anything from simple calculations to complex APIs or external services.
-
Integration with AI Assistants: The MCP server integrates with AI assistants like Claude, allowing the assistant to utilize the exposed tools and functionalities seamlessly within its interactions.
-
Extensibility: By creating an MCP server, you can build custom tools and services tailored to your specific needs, expanding the capabilities of the AI assistant to suit your requirements.
-
Standardized Communication: The MCP protocol provides a standardized way for the AI assistant to communicate with the exposed tools, ensuring a consistent and reliable integration.
Overall, an MCP server enables you to enhance the capabilities of your AI assistant by integrating custom tools and services, allowing you to create a more personalized and powerful AI-driven experience.
Setting up the MCP Server Environment
Setting up the MCP Server Environment
To set up the MCP server environment, follow these steps:
- Open your preferred IDE, in this case, Winsurf.
- Open the terminal and create a new folder for the project:
mkdir count_r_server cd count_r_server
- Create a new virtual environment:
python -m venv .venv
- Activate the virtual environment:
- On Windows:
.venv\Scripts\activate
- On macOS/Linux:
source .venv/bin/activate
- On Windows:
- Install the MCP library:
pip install mcp
- Create a new file named
server.py
to hold the MCP server code.
With the environment set up, you can now proceed to write the MCP server code in the server.py
file.
Creating the MCP Server
Creating the MCP Server
To create a simple MCP (Micro Control Plane) server with a tool that counts the number of 'R's in a given word, follow these steps:
- Open your preferred IDE and create a new folder for the project, e.g.,
count_r_server
. - Navigate to the project folder in the terminal and create a new virtual environment using
python -m venv .venv
. - Activate the virtual environment:
- On Windows:
.venv\Scripts\activate
- On macOS/Linux:
source .venv/bin/activate
- On Windows:
- Install the
mcp
library usingpip install mcp
. - Create a new file named
server.py
and add the following code:
from mcp.server.fast_mcp import FastMCP
import time
import signal
import sys
# Signal handler for graceful shutdown
def signal_handler(sig, frame):
print('Shutting down MCP server...')
server.stop()
sys.exit(0)
# Create the MCP server
server = FastMCP(
name='count_r',
host_url='http://localhost:5000',
timeout=30
)
# Define the 'count_r' tool
@server.mcp_do_tool()
def count_r(word: str) -> int:
try:
if not isinstance(word, str):
return word
return word.lower().count('r')
except Exception as e:
return e
if __name__ == '__main__':
signal.signal(signal.SIGINT, signal_handler)
print('Starting the MCP server...')
server.run()
- Run the server using
python server.py
. You should see the message "Starting the MCP server..." printed to the console.
Now that the MCP server is running, you can integrate it with the Claude desktop application. Follow these steps:
- Open the Claude desktop application and navigate to the "Developer" section in the settings.
- Click the "Edit config" button to open the
config.js
file. - Add the following configuration to the
mcp_servers
section:
{
"name": "count_r",
"command": "python",
"args": [
"/path/to/your/server.py"
],
"host": "http://localhost",
"port": 5000,
"timeout": 30
}
- Save the
config.js
file and restart the Claude desktop application. - You should now see the "count_r" tool available in the Claude desktop application. You can use it to count the number of 'R's in a given word.
Configuring the MCP Server in Claude Desktop
Configuring the MCP Server in Claude Desktop
To configure the MCP server in Claude Desktop, follow these steps:
-
Open the Claude Desktop config.js file by going to the hamburger menu, selecting "File", then "Settings", and clicking on the "Edit config" button under the "Developer" section.
-
In the config.js file, add the following configuration for the MCP server:
"mcp_servers": [
{
"name": "count_r",
"command": "python",
"args": [
"/absolute/path/to/server.py"
],
"host": "localhost",
"port": 5000,
"timeout": 30
}
]
Replace /absolute/path/to/server.py
with the actual file path to your server.py
file.
-
Save the config.js file and restart Claude Desktop.
-
After the restart, you should see the "count_r" tool available in the Claude Desktop interface. You can now use the tool by selecting it and providing the necessary input.
Note that if you don't see the MCP server in Claude Desktop after restarting, you may need to uninstall and reinstall Claude Desktop for the changes to take effect.
Using the MCP Server Tool in Claude Desktop
Using the MCP Server Tool in Claude Desktop
To use the MCP server tool in Claude Desktop, follow these steps:
- Open your IDE of choice, such as Winsurf, and create a new folder for your MCP server project.
- Initialize a new Python virtual environment in the project folder using
python -m venv .venv
. - Activate the virtual environment:
- On Windows:
.venv\Scripts\activate
- On macOS/Linux:
source .venv/bin/activate
- On Windows:
- Install the MCP library using
pip install mcp
. - Create a new file named
server.py
and add the following code:
from mcp.server.fast_mcp import FastMCP
import time
import signal
import sys
# Signal handler for graceful shutdown
def signal_handler(sig, frame):
print('Shutting down MCP server...')
server.stop()
sys.exit(0)
# Set up the MCP server
server = FastMCP(
name='count_r',
host_url='http://localhost:5000',
timeout=30
)
# Define the tool
@server.mcp_do_tool()
def count(word: str) -> int:
try:
if not isinstance(word, str):
return word
return word.lower().count('r')
except Exception as e:
return e
# Start the server
if __name__ == '__main__':
signal.signal(signal.SIGINT, signal_handler)
print('Starting the MCP server...')
server.run()
- Start the MCP server by running
python server.py
in the terminal. - In the Claude Desktop configuration file (
config.js
), add the following configuration:
{
"mcp_servers": [
{
"name": "count_r",
"command": "python",
"args": [
"/path/to/your/server.py"
],
"host": "http://localhost",
"port": 5000,
"timeout": 30
}
]
}
- Restart Claude Desktop, and you should see the "count_r" tool available in the tools menu.
- To use the tool, simply type "use the count_r tool to count the number of Rs in the word strawberry" in the chat, and Claude Desktop will execute the tool and display the result.
Conclusion
Conclusion
Here is the section body in Markdown format:
In this tutorial, we have walked through the process of setting up a simple mCP server using a tool that counts the number of 'R' letters in a given word. We have covered the following steps:
- Creating a new folder and setting up a virtual environment.
- Installing the mCP library and creating the server.py file.
- Defining the mCP tool and handling any exceptions.
- Starting the mCP server and configuring the Claude desktop to recognize it.
The key takeaways from this exercise are:
- mCP servers provide a way to expose tools and APIs to your AI assistant or agent.
- The setup process involves creating a virtual environment, installing the mCP library, and defining the server and tool.
- Configuring the Claude desktop to recognize the mCP server is crucial, and may require uninstalling and reinstalling the desktop application.
- The mCP ecosystem offers a wide range of pre-built servers, which can be found in the "awesome-mcp-servers" GitHub repository.
By following this guide, you should now have a solid understanding of how to set up a simple mCP server and integrate it with the Claude desktop. This knowledge can be applied to building more complex tools and exposing them to your AI assistant.
FAQ
FAQ