使用 ChatGPT 和 Python for VS Code 自動化編碼協助
利用 ChatGPT 和 Python 的力量,為 VS Code 建立一個自訂的程式碼助手。在您的 IDE 中自動化解決方案、回答問題,並提高生產力。
2025年3月25日
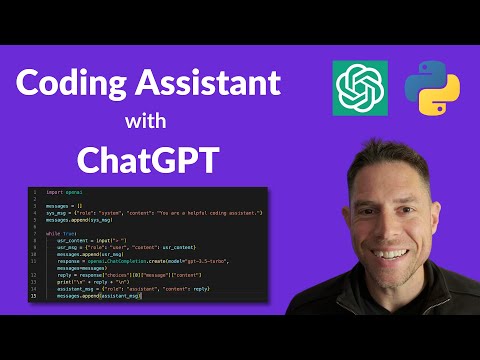
解鎖 ChatGPT 和 Python 的力量,打造您自己的個人化編碼助手。本篇部落格文章將引導您完成創建一個多功能工具的過程,該工具可以在您的開發環境中回答問題並提供見解,提高您的生產力和問題解決能力。
如何使用 ChatGPT 和 Python 建立您自己的編碼助手
設置環境並導入所需的函式庫
初始化聊天歷史記錄
收集使用者輸入並構建訊息
與 ChatGPT API 互動並獲取回應
顯示助手的回應並更新聊天歷史記錄
結論
如何使用 ChatGPT 和 Python 建立您自己的編碼助手
如何使用 ChatGPT 和 Python 建立您自己的編碼助手
要使用 ChatGPT API 和 Python 建立您自己的編碼助手,請遵循以下步驟:
- 將您的 API 金鑰設定為環境變數。
- 安裝 OpenAI 庫並在您的程式碼頂部匯入它。
- 建立一個空白的訊息列表,代表聊天歷史。每個訊息都是一個具有兩個屬性的字典:「角色」和「內容」。
- 將系統訊息附加到聊天歷史,為助手提供靜默指示。
- 設置一個循環,使用
input()
收集來自用戶的新訊息。 - 構建一個新的訊息字典,包含用戶的輸入,並將其附加到聊天歷史。
- 向聊天完成端點發出 OpenAI API 調用,傳遞模型 (
GPT-3.5-turbo
) 和聊天歷史。 - 從 API 響應中提取助手回覆的內容,並將其存儲在變數中。
- 打印助手的回覆,在開頭和結尾添加幾個新行。
- 構建一個新的訊息字典,包含助手的回覆,並將其附加到聊天歷史。
- 重複此過程,允許用戶繼續與編碼助手互動。
這種設置提供了一個簡單而強大的編碼助手,您可以在 VS Code 中運行它,允許您直接在開發環境中獲得有關您的代碼的答案和見解。
設置環境並導入所需的函式庫
設置環境並導入所需的函式庫
要設置環境並導入所需的庫,請遵循以下步驟:
-
將您的 OpenAI API 金鑰設定為環境變數。這可確保您的 API 金鑰保持安全,不會在您的代碼中暴露。
-
使用 pip 安裝
openai
庫:pip install openai
-
在您的代碼頂部導入
openai
庫:import openai
通過這些步驟,您已成功設置環境並導入了與 ChatGPT API 交互所需的庫。
初始化聊天歷史記錄
初始化聊天歷史記錄
要初始化聊天歷史,我們首先創建一個名為 `messages` 的空白列表。此列表將存儲用戶和 AI 助手之間的對話歷史。
聊天歷史中的每條消息都表示為一個具有兩個鍵的字典:「角色」和「內容」。「角色」鍵指示消息的來源,可以是「系統」(用於系統指示)或「用戶」(用於用戶輸入)。「內容」鍵存儲實際的消息文本。
我們從向 `messages` 列表添加一條「系統」消息開始。此消息為 AI 助手提供靜默指示,為對話提供上下文或指導。
```python
messages = []
system_message = {
"role": "system",
"content": "You are a helpful coding assistant."
}
messages.append(system_message)
初始化聊天歷史後,我們可以繼續進入程序的主循環,讓用戶與 AI 助手進行互動。
收集使用者輸入並構建訊息
收集使用者輸入並構建訊息
要收集用戶輸入並構建消息,我們遵循以下步驟:
- 創建一個空白列表來存儲聊天歷史。
- 構建一條「系統」消息作為聊天歷史中的第一條消息。此消息為助手提供靜默指示。
- 設置一個將無限運行的循環。
- 在循環內部,使用
input()
函數收集來自用戶的新消息。 - 構建一個新的消息字典,其中包含用戶的輸入,並將「角色」設置為「用戶」。
- 將新的用戶消息附加到聊天歷史列表。
這個過程允許我們建立對話的上下文,這將在我們呼叫 OpenAI API 生成響應時傳遞。
與 ChatGPT API 互動並獲取回應
與 ChatGPT API 互動並獲取回應
要與 ChatGPT API 交互並檢索響應,我們將遵循以下步驟:
- 將 OpenAI API 金鑰設置為環境變數。
- 安裝 OpenAI 庫並在代碼頂部導入它。
- 創建一個空白列表來存儲聊天歷史,其中每條消息都表示為一個具有「角色」和「內容」鍵的字典。
- 將一條「系統」消息附加到聊天歷史,為助手提供靜默指示。
- 設置一個循環,持續收集用戶輸入並將其作為「用戶」消息添加到聊天歷史。
- 向 ChatGPT 完成端點發出 API 調用,將聊天歷史作為輸入傳遞。
- 從 API 響應中提取助手響應的內容,並將其存儲在變數中。
- 打印助手的響應,在開頭和結尾添加一些新行以提高可讀性。
- 構建一個新的「助手」消息,其中包含響應內容,並將其附加到聊天歷史。
- 重複此過程,允許用戶繼續與編碼助手互動。
顯示助手的回應並更新聊天歷史記錄
顯示助手的回應並更新聊天歷史記錄
要顯示助手的響應並更新聊天歷史,我們遵循以下步驟:
1. 從 API 調用中檢索助手響應的內容:
```python
reply = response.choices[0].message.content
-
使用一些格式化打印助手的響應:
print("\n\n" + reply + "\n\n")
-
構建一個新的消息字典來表示助手的響應:
assistant_message = { "role": "assistant", "content": reply }
-
將助手的消息附加到聊天歷史:
messages.append(assistant_message)
這確保了助手的響應以清晰和可讀的格式顯示,並且聊天歷史已更新為包含來自助手的新消息。更新後的聊天歷史將在後續的 API 調用中用作上下文,以提供助手的響應。
結論
結論
在本教程中,我們學習了如何使用 ChatGPT API 和 Python 構建強大的編碼助手。最終產品允許您在 VS Code 中運行該腳本,為您提供一個有用的助手,可以回答有關您正在編寫的代碼的問題並提供見解。
構建此助手的關鍵步驟包括:
- 將 API 金鑰設置為環境變數。
- 安裝 OpenAI 庫並將其導入到您的 Python 腳本中。
- 創建一個消息列表來表示聊天歷史,從一條系統消息開始。
- 實現一個循環,持續收集用戶輸入並將其附加到聊天歷史。
- 向 ChatGPT 完成端點發出 API 調用,傳遞聊天歷史。
- 從 API 中提取響應,並將其作為助手消息附加到聊天歷史。
通過遵循這個過程,您可以輕鬆地將強大的 AI 驅動的編碼助手集成到您的開發工作流程中,解鎖新的生產力和效率水平。
常問問題
常問問題