Unleash Multi-Agent Assistants with Experts.js: Streamlining OpenAI API Usage
Unleash the power of multi-agent AI systems with Experts.js, the streamlined way to use the OpenAI API. Discover how to create a panel of specialized AI experts, enhance performance, and save tokens.
June 27, 2025
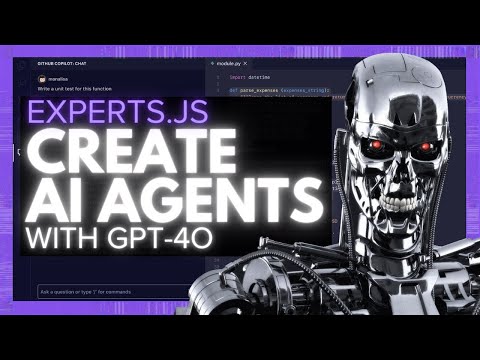
Experts.js is a powerful multi-agent framework that simplifies the use of OpenAI's Assistant API, allowing you to create and deploy specialized AI assistants that work together seamlessly. This framework offers enhanced memory, refined generation, and attention to detail, providing you with a more contextual and efficient AI experience. Whether you're a developer or a business looking to leverage the power of AI, Experts.js offers a modular and streamlined approach to building intelligent systems.
Simplify and Streamline Your AI Assistant Development with Experts.js
Unlock the Power of Multi-Agent Systems with Experts.js
Efficient and Specialized Assistants: Experts.js Architecture
Get Started with Experts.js: Installation and Usage
Create and Configure Your Assistants with Experts.js
Leverage Open AI's Tools and Function Calls with Experts.js
Conclusion
Simplify and Streamline Your AI Assistant Development with Experts.js
Simplify and Streamline Your AI Assistant Development with Experts.js
Experts.js is a powerful library designed to simplify the usage of OpenAI's Assistant API. It offers a multi-agent system that allows you to create a panel of specialized AI assistants, each focused on a specific domain or context.
The key benefits of using Experts.js include:
-
Simplicity and Ease of Use: Experts.js removes the complexity of managing run objects, making it easier for anyone to understand and work with.
-
Multi-Agent System: Experts.js enables you to create a panel of AI experts, each specializing in their own domain. These assistants are automatically linked together as tools, allowing them to work cohesively.
-
Modular Approach: The architecture of Experts.js promotes a modular design, where each assistant is focused on a specific task, preventing overlap and token wastage.
-
Thread Management: Experts.js handles the creation and management of threads automatically, ensuring that multiple tools can work simultaneously without causing issues.
To get started with Experts.js, follow these steps:
- Install the library via npm:
npm install experts.js
- Import the necessary components:
import { Assistant, Tools, Threads } from 'experts.js'
- Create your assistant by extending the
Assistant
class and configuring it with the desired model and tool set. - Utilize the various tools and function calls provided by OpenAI, which can be accessed through the Experts.js interface.
- Explore the advanced features, such as integrating third-party tools, to further enhance your AI assistant development.
By leveraging Experts.js, you can streamline your AI assistant development process, create a modular and efficient system, and take advantage of the powerful capabilities offered by OpenAI's Assistant API.
Unlock the Power of Multi-Agent Systems with Experts.js
Unlock the Power of Multi-Agent Systems with Experts.js
Experts.js is a powerful library designed to simplify the usage of OpenAI's Assistant API. It introduces a multi-agent system that allows you to create a panel of specialized AI assistants, each focused on a specific domain or context.
The key benefits of Experts.js include:
-
Simplicity and Ease of Use: Experts.js removes the complexity of managing run objects, making it easy for anyone to build powerful AI systems.
-
Multi-Agent System: Experts.js enables you to create a panel of AI experts, each specializing in a particular domain. These experts are automatically linked together as tools, allowing them to work cohesively.
-
Modular Approach: The multi-agent system in Experts.js ensures that each assistant is focused on its specific task, preventing overlap and token wastage. This enhances the overall performance and efficiency of the system.
-
Thread Management: Experts.js handles the creation and management of threads automatically, allowing you to focus on building your AI applications without worrying about extra IDs on the client-side.
To get started with Experts.js, simply follow the installation instructions on the GitHub repository. Once set up, you can create your assistant, define the tools, and leverage the various features provided by the library, such as function calls, streaming, and integration with third-party tools.
By unlocking the power of multi-agent systems with Experts.js, you can build more specialized, efficient, and scalable AI applications that cater to your specific needs.
Efficient and Specialized Assistants: Experts.js Architecture
Efficient and Specialized Assistants: Experts.js Architecture
Experts.js is a library designed to streamline the use of OpenAI's Assistant API. It introduces a multi-agent system that allows you to create a panel of AI experts, each specializing in a specific domain and context. This modular approach ensures that each assistant is focused on its own task, preventing overlap and token wastage.
The architecture showcases a practical use case where a primary sales and routing assistant utilizes specialized tools, such as a merchandising expert and an open search tool. This setup allows the sales assistant to focus on its core responsibilities, delegating complex tasks to the appropriate experts. The open search tool, for instance, handles all the queries related to the open search vector database, without the sales assistant needing to know how to perform these operations.
This approach keeps the assistants efficient and specialized, enhancing overall performance and preventing confusion. It also helps in saving tokens by ensuring that each assistant only performs the tasks it is best suited for.
Another key feature of Experts.js is its thread management. Each tool runs on its own space, preventing problems when multiple tools need to work simultaneously. The library automatically creates and manages these threads, so you don't have to worry about extra IDs on the client-side.
To get started with Experts.js, you can follow the installation instructions on the GitHub repository. The usage is straightforward, involving the creation of assistants, tools, and threads. You can leverage OpenAI's existing tools and function calls, as well as create your own custom tools to suit your specific needs.
Get Started with Experts.js: Installation and Usage
Get Started with Experts.js: Installation and Usage
To get started with Experts.js, follow these steps:
-
Installation: Go to the GitHub repository and scroll down to the "Installation" tab. You can install Experts.js via npm by running the following command in your terminal:
npm install experts.js
-
Usage: After installing the package, you can import the necessary components and start using Experts.js. Here's an example:
import { Assistant, Tools, Threads } from 'experts.js'; // Create your assistant const assistant = new Assistant({ name: 'My Assistant', description: 'A multi-agent AI assistant', instruction: 'I am an AI assistant here to help you with various tasks.', model: 'gpt-4-turbo', tools: [/* your tools */] }); // Create your tools const searchTool = new Tools.OpenSearchTool({ name: 'Open Search', description: 'A tool for searching open data sources' }); // Create a thread to manage the context const thread = new Threads.Thread(); // Ask your assistant a question const response = await assistant.ask('What is the capital of France?', { thread }); console.log(response);
-
Explore Further: Experts.js provides a modular and extensible approach to building multi-agent AI systems. Be sure to read through the documentation to learn more about creating custom tools, integrating third-party services, and leveraging advanced features like streaming and events.
The key benefits of using Experts.js include:
- Simplicity and Ease of Use: Experts.js abstracts away the complexity of managing run objects, making it easier to create and deploy OpenAI-powered assistants.
- Multi-Agent System: Experts.js allows you to create a panel of specialized AI agents that work together as tools, enhancing the overall performance and efficiency.
- Thread Management: Experts.js automatically handles thread management, ensuring that each tool runs in its own space without conflicts.
By following the steps outlined above, you can get started with Experts.js and start building powerful multi-agent AI systems for your applications.
Create and Configure Your Assistants with Experts.js
Create and Configure Your Assistants with Experts.js
To get started with Experts.js, you first need to create your assistant. This is where the assistant represents the AI agent, and you need to create it by defining a new assistant and extending the Assistant
class.
Here's an example of how you can set up your assistant:
import { Assistant } from 'experts.js';
const myAssistant = new Assistant({
name: 'My Assistant',
description: 'A versatile AI assistant',
instruction: 'You are a helpful and knowledgeable AI assistant. Please assist me with any tasks or questions I have.',
model: 'gpt-4-turbo',
tools: ['code-interpreter', 'file-search']
});
In this example, we're creating a new assistant with a name, description, and instruction. We're also configuring it to use the gpt-4-turbo
model and providing it with the code-interpreter
and file-search
tools.
You can also utilize all of OpenAI's tools and function calling features, which you can access through the OpenAI documentation. This allows you to integrate various capabilities into your assistant, such as code interpretation, file search, and more.
To interact with your assistant, you can use the ask
interface, which allows you to instruct your assistant to perform specific tasks:
const response = await myAssistant.ask('Can you summarize the key points of this document?');
console.log(response);
Experts.js also supports streaming and events, allowing you to work with advanced features and integrate third-party tools as needed. Be sure to review the Experts.js documentation to learn more about the available features and how to create a multi-agent system with interconnected assistants.
Leverage Open AI's Tools and Function Calls with Experts.js
Leverage Open AI's Tools and Function Calls with Experts.js
One of the key features of Experts.js is its ability to seamlessly integrate with Open AI's tools and function calls. This allows you to leverage the powerful capabilities of the Open AI Assistant API within your Experts.js-based multi-agent system.
Through Experts.js, you can access a wide range of Open AI tools and function calls, including:
- Code Interpreter
- File Search
- And many other tools listed in the Open AI documentation
To utilize these features, you can simply add the desired tools to your Experts.js assistant configuration. This will enable your assistant to call upon these specialized tools as needed, ensuring efficient and focused task execution.
Furthermore, Experts.js provides a straightforward interface for interacting with your assistant, allowing you to ask questions and provide instructions. The system will then seamlessly delegate tasks to the appropriate tools and agents, leveraging the power of Open AI's capabilities.
By combining the flexibility and modularity of Experts.js with the robust functionality of Open AI's tools and function calls, you can create highly capable and specialized multi-agent systems tailored to your specific needs. This integration empowers you to build powerful AI-driven applications with ease.
Conclusion
Conclusion
The introduction of Experts.js is a significant development in the world of AI-powered applications. This library simplifies the usage of OpenAI's Assistant API, making it easier for developers to create and deploy powerful AI systems.
The key highlights of Experts.js include:
-
Simplicity and Ease of Use: Experts.js removes the complexity of managing run objects, allowing developers to focus on building their AI applications.
-
Multi-Agent System: Experts.js introduces a modular approach, enabling the creation of a panel of specialized AI assistants that work cohesively as tools. This ensures each assistant is focused on a specific domain, enhancing efficiency and preventing token wastage.
-
Thread Management: Experts.js automatically handles thread management, allowing multiple tools to work simultaneously without causing problems.
To get started with Experts.js, developers can follow the installation and usage instructions provided in the GitHub repository. By leveraging the power of OpenAI's tools and function calls, developers can create advanced AI-powered applications with ease.
Overall, Experts.js is a valuable addition to the AI development ecosystem, simplifying the process of building and deploying AI assistants and unlocking new possibilities for innovative applications.
FAQ
FAQ